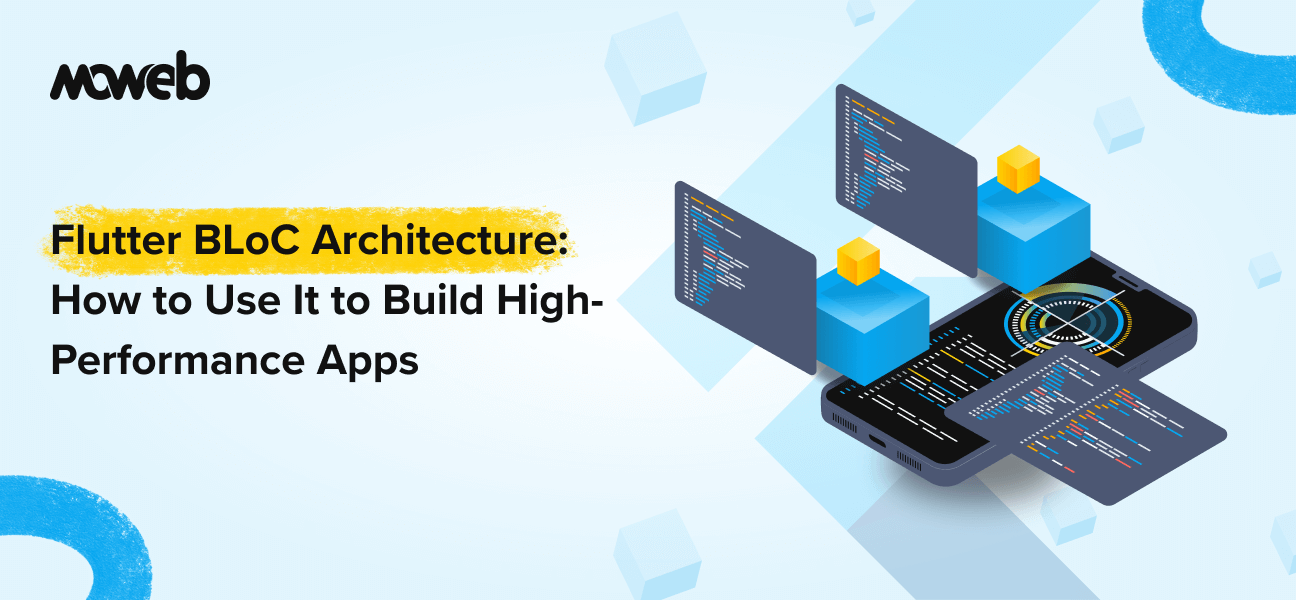
In the dynamic world of mobile app development, architecture is more than just code—it’s the foundation that determines your app’s scalability, performance, and user experience. One of the most effective architectural patterns for building scalable and high-performing apps in the Flutter framework is the BLoC (Business Logic Component) architecture.
The BLoC architecture empowers developers to create clean, organized, and reactive Flutter applications by effectively separating UI from business logic. This structured approach not only simplifies development but also ensures apps are easier to test, maintain, and scale.
In this blog, we’ll explore the essentials of Flutter BLoC Architecture, why it’s a go-to choice for developers, and how it elevates app development to the next level. From understanding its core components—like events, states, and streams—to implementing it in real-world projects, you’ll gain actionable insights to build Flutter apps that perform seamlessly across platforms.
Ready to discover how Flutter BLoC can revolutionize your app development?
What is Flutter BLoC Architecture?
In Flutter development, the BLoC (Business Logic Component) architecture is a design pattern that promotes a clean separation between the user interface (UI) and the business logic of an app. Its primary purpose is to organize your codebase efficiently, ensuring scalability and maintainability. This makes BLoC architecture a popular choice for developers aiming to create robust, high-performance cross-platform applications.
Why Do Developers Need Structured Architecture?
Structured architecture, like BLoC, is essential in Flutter projects to prevent code from becoming tangled and difficult to manage. By clearly defining responsibilities within the app, developers can:
- Enhance code readability and reusability.
- Reduce the likelihood of bugs and performance issues.
- Simplify testing processes for individual components.
The Role of BLoC in Flutter Development
At its core, BLoC acts as the mediator between the UI and the app’s business logic. Instead of directly embedding logic into UI components, BLoC captures events triggered by the UI, processes them, and returns the corresponding state. This approach creates a reactive framework where the UI updates dynamically based on state changes, making apps not only more efficient but also easier to scale.
By leveraging streams to manage event-state communication, Flutter BLoC architecture ensures smooth data flow and clean interaction between components, fostering a consistent and responsive user experience.
Key Components of Flutter BLoC Architecture
Flutter BLoC Architecture consists of several integral components that work together to create a seamless and efficient structure for app development. By enabling clear separation between the UI and business logic, these components form the backbone of a clean, scalable, and maintainable Flutter application. Let’s delve into the key components:
- BLoC (Business Logic Component)
The BLoC serves as the intermediary layer between the UI and data layers. It processes input events from the UI, performs the necessary business logic, and emits corresponding states for the UI to render. By isolating the logic from the UI, the BLoC ensures a more modular and testable codebase. - Streams
Streams are the core mechanism enabling reactive programming in Flutter BLoC Architecture. They provide real-time communication by allowing the UI to listen for state changes and react accordingly. With streams, data flows seamlessly between events and states, making it easier to implement dynamic user interfaces. - Events
Events are the triggers that initiate changes in the application. They represent user actions or system updates, such as button clicks, form submissions, or API responses. Each event is captured by the BLoC, where it is processed to determine the corresponding app state. - States
States are the outcomes of processing events within the BLoC. They represent the app’s current state and dictate how the UI should render. For example, states can indicate loading screens, success messages, or error notifications, ensuring the app dynamically reflects changes. - Cubit (Optional Simplified Approach)
Cubit is a lightweight alternative to BLoC that simplifies state management by eliminating the need for events. It focuses purely on emitting states, making it ideal for smaller, less complex Flutter projects while retaining the core principles of reactive programming.
Flutter BLoC (Business Logic Component) Design Pattern
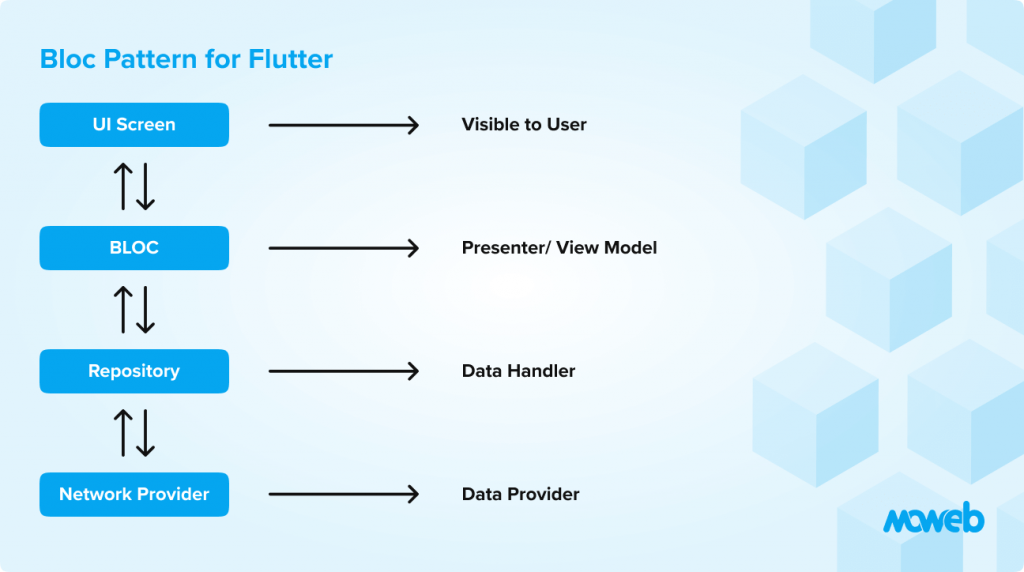
Interaction Between Components
The interaction between these components creates a seamless event-driven architecture:
- User Interaction: The user triggers an action (e.g., clicking a button), which generates an event.
- Event Processing: The event is captured by the BLoC, which processes it using defined business logic.
- State Emission: After processing, the BLoC emits a new state.
- UI Update: The UI listens to the state stream and updates itself accordingly.
This architecture ensures that each layer operates independently, promoting maintainability and scalability.
Want to know more about Flutter architecture patterns? Check out our Comprehensive Flutter App Development Guide.
Understanding BLoC Concepts: Events and States
The BLoC Architecture in Flutter revolves around two fundamental concepts: Events and States. These concepts enable the smooth flow of data between the UI and the business logic, making the architecture reactive and intuitive.
Events: What Are They and How Are They Triggered?
Events represent user actions or system-generated inputs that trigger changes in the application. For example, actions like a button click, text input, or an API call response are translated into specific events.
- Triggering Events: Events are triggered through user interactions or external factors, such as network responses or system notifications.
- Role in BLoC: Events are captured by the BLoC layer, where the business logic processes them to determine the next course of action, ensuring a well-structured flow of functionality.
For example:- Clicking a “Login” button generates a LoginEvent.
- Scrolling through a feed could trigger a LoadMorePostsEvent.
States: How Do They Represent the App’s Dynamic Behavior?
States are the outputs of the BLoC layer, representing the app’s current condition after processing events. They dictate what the user sees and interacts with on the screen.
- Dynamic Behavior: States allow the app to dynamically update its UI based on changes, such as displaying a loading spinner during a data fetch or showing a success message after a form submission.
- Role in Flutter Apps: Each state ensures the app transitions smoothly between different visual or functional stages, enhancing the user experience.
For example:- A LoadingState could display a progress indicator.
- A LoadedState could display fetched data.
- An ErrorState might show an error message if something goes wrong.
Benefits of Flutter BLoC Architecture
The Flutter BLoC Architecture offers several advantages, making it a preferred choice for developers aiming to create clean, scalable, and maintainable apps
- Ensures Separation of Concerns
By separating the UI from the business logic, BLoC promotes cleaner, more modular code. This separation makes the app easier to develop, test, and debug. - Facilitates Reusability of Business Logic
The business logic in the BLoC layer is not tied to a specific UI, allowing developers to reuse the same logic across multiple screens or even apps. - Improves Testability
The structured architecture ensures that the logic and UI are independently testable, allowing developers to write unit tests for the business logic without involving the UI. - Scalability for Complex Apps
BLoC’s event-state management system makes it easier to scale apps, accommodating more features and complex user interactions without compromising performance. - Simplifies Maintenance
With its clear organization of components, BLoC simplifies the process of maintaining and updating the app, ensuring long-term sustainability. - Supports Reactive Programming
Using streams enables real-time updates, ensuring the app reacts seamlessly to changes in user inputs or external data.
Related post: Flutter a Good Choice for Cross-Platform App Development
How to Use BLoC Architecture in Flutter to Build Apps
Implementing Flutter BLoC Architecture in your app development project involves understanding its fundamental concepts and setting up the necessary components for smooth event-state management. Here’s a step-by-step guide to using the BLoC pattern effectively in Flutter:
- Setting Up Your Flutter Project
Before diving into the implementation, it’s important to set up your Flutter environment:
Install the required dependencies such as flutter_bloc and bloc_test by adding them to your pubspec.yaml file:
yaml
CopyEdit
dependencies:
flutter_bloc: ^8.0.0
bloc: ^8.0.0
dev_dependencies:
Run the flutter pub get command to fetch the packages and ensure they’re ready for use in your project.
- Creating a BLoC Class
- At the core of the Flutter BLoC Architecture is the BLoC class, where the business logic resides. Here’s how to create one:
Define Events: List all the possible user interactions or actions that the BLoC will handle.
dart
CopyEdit
abstract class CounterEvent {}
class IncrementEvent extends CounterEvent {}
class DecrementEvent extends CounterEvent {}
Define States: Represent the various UI conditions based on user actions or events.
dart
CopyEdit
abstract class CounterState {}
class CounterInitial extends CounterState {}
class CounterValue extends CounterState {
final int value;
CounterValue(this.value);
}
- Write the BLoC Logic: Use streams to process events and emit corresponding states.
dart
CopyEdit
class CounterBloc extends Bloc<CounterEvent, CounterState> {
CounterBloc() : super(CounterInitial()) {
on<IncrementEvent>((event, emit) {
// Logic for incrementing the counter
});
on<DecrementEvent>((event, emit) {
// Logic for decrementing the counter
});
}
}
3. Implementing Streams for Event-State Management
Streams play a crucial role in BLoC architecture by enabling reactive data flow:
- Listen to Events: The BLoC listens for incoming events and processes them.
- Emit States: Based on the processed events, the BLoC emits new states, which are reflected in the UI. For example:
dart
CopyEdit
void _mapIncrementToState(IncrementEvent event, Emitter<CounterState> emit) {
final currentValue = (state is CounterValue) ? (state as CounterValue).value : 0;
emit(CounterValue(currentValue + 1));
}
4. Integrating BLoC with UI
Use Flutter widgets like BlocProvider and BlocBuilder to connect the BLoC logic with the UI:
- BlocProvider: Wrap the widget tree with a BlocProvider to inject the BLoC instance:
dart
CopyEdit
BlocProvider(
create: (context) => CounterBloc(),
child: CounterScreen(),
);
- BlocBuilder: Listen to state changes and rebuild the UI accordingly:
dart
CopyEdit
BlocBuilder<CounterBloc, CounterState>(
builder: (context, state) {
if (state is CounterValue) {
return Text(‘Counter: ${state.value}’);
}
return Text(‘Counter: 0’);
},
);
5. Testing Your BLoC Logic
Ensure your BLoC implementation works as expected by writing unit tests using bloc_test:
- Verify the correct state is emitted for a given event.
dart
CopyEdit
blocTest<CounterBloc, CounterState>(
‘ emits [CounterValue] when IncrementEvent is added’,
build: () => CounterBloc(),
act: (bloc) => bloc.add(IncrementEvent()),
expect: () => [CounterValue(1)],
);
Why BLoC is a Preferred Architecture in Flutter
The BLoC pattern is an ideal choice for building scalable and high-performing apps, whether you’re working on an e-commerce platform, enterprise application, or on-demand service. By separating the UI from the business logic, it ensures a clean architecture that enhances testability and long-term maintenance.
Related Post: Flutter vs React Native: A Comprehensive Guide
Best Practices for Using Flutter BLoC Architecture
Implementing Flutter BLoC Architecture effectively requires following best practices that ensure clean, maintainable, and scalable app development. Here’s a look at essential practices to follow when working with the BLoC pattern in Flutter projects:
- Adopt the Single Responsibility Principle
Each BLoC class should focus on a single functionality or feature within the app. This practice simplifies debugging, enhances readability, and ensures maintainability as the project scales. Overloading a BLoC with multiple responsibilities can lead to complex code that’s difficult to manage in the long run. - Use Descriptive Names for Events and States
Clear and intuitive naming conventions for events and states improve code readability and make the purpose of each component immediately apparent. For instance
Instead of generic names like Event1 or StateA, use UserLoggedInEvent or LoadingState to convey their functionality. This helps developers understand and extend the codebase without unnecessary guesswork. - Leverage BlocBuilder Efficiently
Use BlocBuilder only for widgets that need to rebuild based on state changes. Overusing it across an entire widget tree can lead to unnecessary rebuilds and impact performance. Instead:
-Wrap specific widgets with BlocBuilder to ensure efficient state-based UI updates.
-Combine it with BlocListener to handle one-time events like showing snack bars or dialog boxes. - Separate Business Logic from Presentation
Ensure your business logic resides within the BLoC and not in the UI layer. This separation maintains a clean architecture and makes the app more testable and flexible for future enhancements. Keeping the UI layer focused solely on rendering ensures modular and reusable components. - Test Your BLoC Logic Thoroughly
Use tools like bloc_test to validate the behavior of your BLoC. Write unit tests for every event-to-state transition, ensuring predictable and reliable outcomes. Comprehensive testing safeguards your app against regressions during future updates. - Optimize Stream Usage
Streams are integral to the BLoC pattern, so ensure they are used optimally:
-Close streams when they’re no longer needed to prevent memory leaks.
-Use .distinct() or .debounceTime() operators when working with frequent or duplicate events to reduce unnecessary processing. - Implement Error Handling
Add proper error-handling mechanisms within the BLoC to manage unexpected issues gracefully. This ensures the app remains robust and user-friendly, even in the face of backend failures or invalid inputs. - How Moweb Can Help
At Moweb Limited, we excel in creating scalable, high-performing apps using Flutter BLoC Architecture. Our experienced team:
-Designs well-structured BLoC implementations adhering to best practices.
-Optimizes app performance with clean and modular architecture.
-Provides robust testing and post-launch support to keep your app competitive in a dynamic market.
Conclusion: Unlock the Power of Flutter BLoC Architecture
The Flutter BLoC Architecture stands out as a game-changing pattern that ensures high-performing, scalable, and maintainable apps. By separating UI from business logic, Flutter’s BLoC framework empowers developers to build robust and seamless applications, making it ideal for startups and enterprises alike. Its structured approach and emphasis on reactive programming simplify complex workflows and accelerate development timelines.
Now is the perfect time to integrate BLoC architecture in Flutter development into your projects. Whether choosing Flutter for MVP development or a complex enterprise app, leveraging this architecture can elevate your app’s performance and ensure it meets user expectations effectively.
Why Choose Moweb Limited?
At Moweb Limited, we specialize in creating innovative Flutter solutions with a strong foundation in BLoC architecture. Our team of experts:
- Designs intuitive and scalable app architectures tailored to business goals.
- Offers end-to-end Flutter app development services, ensuring seamless project execution.
- Provides unmatched post-launch support to keep your app competitive in the dynamic market.
Let’s bring your app idea to life with the expertise and dedication your business deserves.
Ready to get started?
Explore the benefits of Flutter BLoC Architecture with us. Hire Flutter App Developers today and transform your vision into a high-performing reality.
Found this post insightful? Don’t forget to share it with your network!